Hi there
I'm currently running into an issue on a combo box selector form being naughty, so to speak. The purpose of the form is to be able to select a customer or a facility, that is filtered by customers
(Customers and Facilities are a 1 - many with 1 customer to many facilities)
When you select a customer it filters out the facilities you can choose to ONLY facilities related to the customer chosen. This forms purpose is to be able to edit customer or facility records. I want to be able to select JUST a customer (no facility) click continue, and it opens up the associated customer record, or if I choose a Facility I'd want it to open up the associated facility record.
Here are some shots of what the form looks like so you can get a better visual:
ONLY Customer is selected here:
Customer AND Facility are selected here:
My code is as follows:
Private Sub BtnContinue_over_Click()
On Error GoTo Err_BtnContinue_over_Click
Dim stDocCust As String
Dim stDocFac As String
Dim stLinkCriteria As String
Dim CustInt As Integer
Dim FacInt As Integer
stDocCust = "Customers"
stDocFac = "FacilityInfo"
If Me.CustCmbo_Customers <> "" And Me.CustCmbo_Facilities = Null Then
DoCmd.OpenForm stDocCust, , , Me.CustCmbo_Customers = Forms!Customers![Customers-CustName]
ElseIf Me.CustCmbo_Customers <> "" And Me.CustCmbo_Facilities <> "" Then
DoCmd.OpenForm stDocFac, , , Me.CustCmbo_Facilities = Forms!FacilityInfo![FacilityInfo-FacilityName]
End If
Exit_BtnContinue_over_Click:
Exit Sub
Err_BtnContinue_over_Click:
MsgBox Err.Description
Resume Exit_BtnContinue_over_Click
End Sub
This is the code that does the filtering:
Private Sub CustCmbo_Customers_Change()
Dim q As String
Dim sel As Integer
q = "SELECT FacilityID, Facility_Name FROM Facilities WHERE CustomerID = " & Me.[CustCmbo-Customers].Column(0) & " ORDER BY Facility_Name"
Me.[CustCmbo-Facilities].RowSource = q
Me.[CustCmbo-Facilities].BoundColumn = 1
Me.[CustCmbo-Facilities].Requery
End Sub
Any help is apprecitated once again
I'm currently running into an issue on a combo box selector form being naughty, so to speak. The purpose of the form is to be able to select a customer or a facility, that is filtered by customers
(Customers and Facilities are a 1 - many with 1 customer to many facilities)
When you select a customer it filters out the facilities you can choose to ONLY facilities related to the customer chosen. This forms purpose is to be able to edit customer or facility records. I want to be able to select JUST a customer (no facility) click continue, and it opens up the associated customer record, or if I choose a Facility I'd want it to open up the associated facility record.
Here are some shots of what the form looks like so you can get a better visual:
ONLY Customer is selected here:
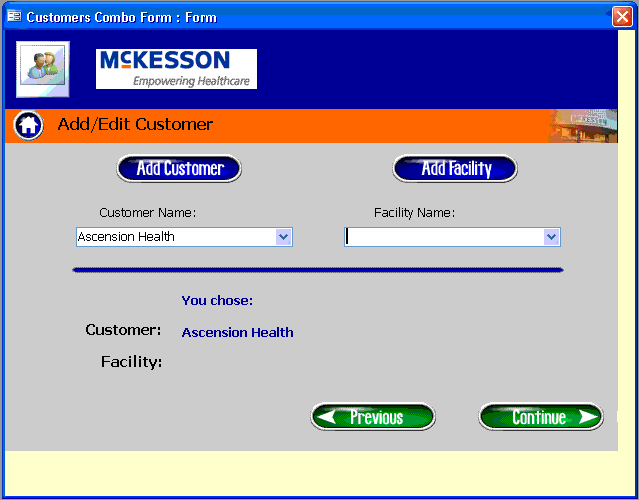
Customer AND Facility are selected here:
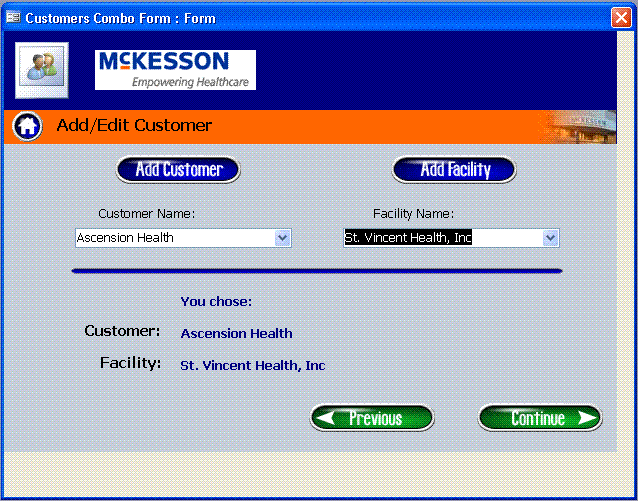
My code is as follows:
Private Sub BtnContinue_over_Click()
On Error GoTo Err_BtnContinue_over_Click
Dim stDocCust As String
Dim stDocFac As String
Dim stLinkCriteria As String
Dim CustInt As Integer
Dim FacInt As Integer
stDocCust = "Customers"
stDocFac = "FacilityInfo"
If Me.CustCmbo_Customers <> "" And Me.CustCmbo_Facilities = Null Then
DoCmd.OpenForm stDocCust, , , Me.CustCmbo_Customers = Forms!Customers![Customers-CustName]
ElseIf Me.CustCmbo_Customers <> "" And Me.CustCmbo_Facilities <> "" Then
DoCmd.OpenForm stDocFac, , , Me.CustCmbo_Facilities = Forms!FacilityInfo![FacilityInfo-FacilityName]
End If
Exit_BtnContinue_over_Click:
Exit Sub
Err_BtnContinue_over_Click:
MsgBox Err.Description
Resume Exit_BtnContinue_over_Click
End Sub
This is the code that does the filtering:
Private Sub CustCmbo_Customers_Change()
Dim q As String
Dim sel As Integer
q = "SELECT FacilityID, Facility_Name FROM Facilities WHERE CustomerID = " & Me.[CustCmbo-Customers].Column(0) & " ORDER BY Facility_Name"
Me.[CustCmbo-Facilities].RowSource = q
Me.[CustCmbo-Facilities].BoundColumn = 1
Me.[CustCmbo-Facilities].Requery
End Sub
Any help is apprecitated once again
Last edited: