peskywinnets
Registered User.
- Local time
- Today, 19:27
- Joined
- Feb 4, 2014
- Messages
- 578
Ok, so I'm trying to knock up an API to connect to Amazon...the API itself isn't the problem, it's the hashing process.
To test everyone's hashing process, Amazon provided some test data to work with.
The process is to start off with a known key, then use that key to hash some data .....the output of which you then use to hash the next piece of data...and so on
so we start off with a signing key of AWS4wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY ...the first thing we want to hash is a sample date of 20120215 therefore
kdate = ComputeHMACSHA256("AWS4wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY", "20120215")
that should produce an output of 969fbb94feb542b71ede6f87fe4d5fa29c789342b0f407474670f0c2489e0a0d ...and this bit does indeed work.
we then use the output to hash another bit of sample region data us-east-1 therefore...
kregion = ComputeHMACSHA256("969fbb94feb542b71ede6f87fe4d5fa29c789342b0f407474670f0c2489e0a0d", "us-east-1")
that should produce an output of 69daa0209cd9c5ff5c8ced464a696fd4252e981430b10e3d3fd8e2f197d7a70c ...but it doesn't
Someone else raised this here...
stackoverflow.com
....and someone did chime in with an answer & the associated code (which produces the correct output), but they are discussing PHP - I'm out my depth and don't know how to deploy in VBA (on stackoverflow, they reference an online hashing tool... https://hash.online-convert.com/sha256-generator )
It seems to hinge on how data is stored vs how it's displayed ...but I've read it way too many times & I'm not getting it.
Here's the simple code if anyone wants a dabble....
ComputeHMACSHA256 = LCase(hmacsha)
End Function
To test everyone's hashing process, Amazon provided some test data to work with.
The process is to start off with a known key, then use that key to hash some data .....the output of which you then use to hash the next piece of data...and so on
so we start off with a signing key of AWS4wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY ...the first thing we want to hash is a sample date of 20120215 therefore
kdate = ComputeHMACSHA256("AWS4wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY", "20120215")
that should produce an output of 969fbb94feb542b71ede6f87fe4d5fa29c789342b0f407474670f0c2489e0a0d ...and this bit does indeed work.
we then use the output to hash another bit of sample region data us-east-1 therefore...
kregion = ComputeHMACSHA256("969fbb94feb542b71ede6f87fe4d5fa29c789342b0f407474670f0c2489e0a0d", "us-east-1")
that should produce an output of 69daa0209cd9c5ff5c8ced464a696fd4252e981430b10e3d3fd8e2f197d7a70c ...but it doesn't
Someone else raised this here...
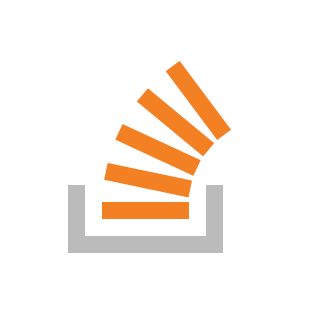
AWS4 Signature key - is this tutorial wrong?
According to this page: Examples of How to Derive a Signing Key for Signature Version 4 The result of this code: $kSecret = "wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY"; $kDate = hash_hmac('sha256...
....and someone did chime in with an answer & the associated code (which produces the correct output), but they are discussing PHP - I'm out my depth and don't know how to deploy in VBA (on stackoverflow, they reference an online hashing tool... https://hash.online-convert.com/sha256-generator )
It seems to hinge on how data is stored vs how it's displayed ...but I've read it way too many times & I'm not getting it.
Here's the simple code if anyone wants a dabble....
Code:
Public Function TestHMAC()
Dim kdate As String
Dim kregion As String
Key = "AWS4wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY"
dateStamp = "20120215"
regionName = "us-east-1"
kdate = ComputeHMACSHA256("AWS4wJalrXUtnFEMI/K7MDENG+bPxRfiCYEXAMPLEKEY", "20120215")
Debug.Print kdate ' should come out as 969fbb94feb542b71ede6f87fe4d5fa29c789342b0f407474670f0c2489e0a0d
kregion = ComputeHMACSHA256(kdate, "20120215")
End Function
Function ComputeHMACSHA256(Key As String, Text As String) As String
Dim encoder As Object, crypto As Object
Dim hash() As Byte, hmacsha As String, i As Long
' compute HMACSHA256
Set encoder = CreateObject("System.Text.UTF8Encoding")
Set crypto = CreateObject("System.Security.Cryptography.HMACSHA256")
crypto.Key = encoder.GetBytes_4(Key)
hash = crypto.ComputeHash_2(encoder.GetBytes_4(Text))
' convert to an hexa string
hmacsha = String(64, "0")
For i = 0 To 31
Mid$(hmacsha, i + i + (hash(i) > 15) + 2) = Hex(hash(i))
Next
ComputeHMACSHA256 = LCase(hmacsha)
End Function