Hi All
I know this is a big ask, and so many have helped me to date, hopefully someone can help.
on our database we produce passwords using the website:
bitwarden.com
I have a button called BTNPassGenerator, when the user clicks on this ideally the following process needs to be done
> Open website https://bitwarden.com/password-generator (in edge or chrome)
>Type - Passphrase
> Words: 3
> Capitalize - YES
> Include Number - YES
Once this is done then copies the password and Pastes into back into the Access textbox TXTPassword
Thanks in advance!
Stu
I know this is a big ask, and so many have helped me to date, hopefully someone can help.
on our database we produce passwords using the website:
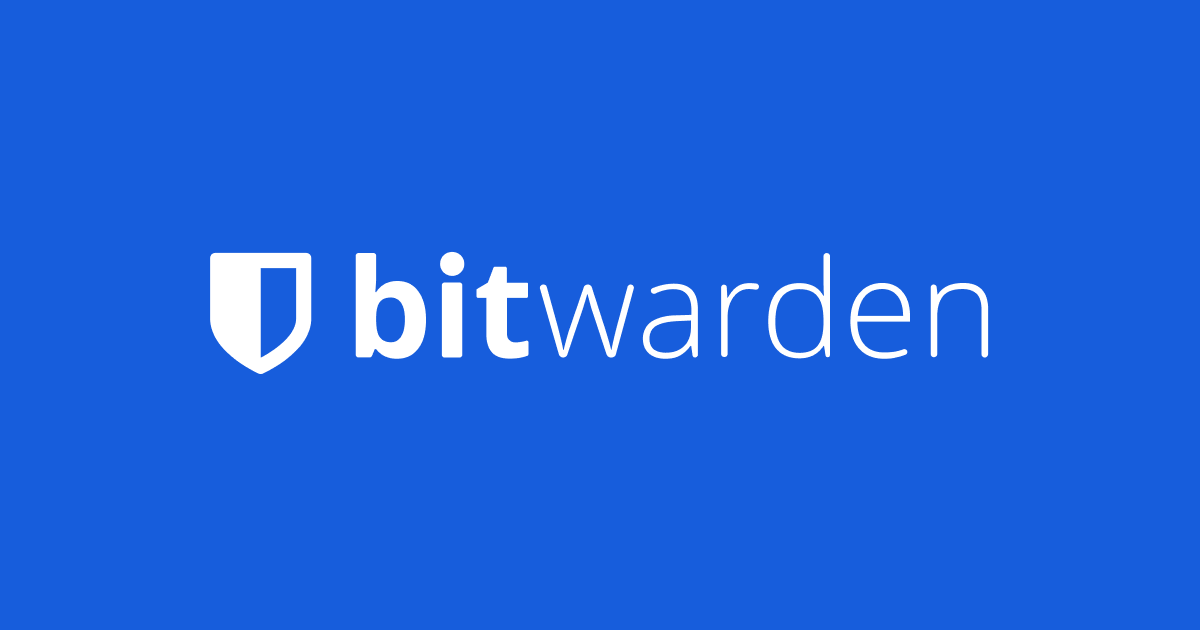
Free Password Generator | Create Strong Passwords | Bitwarden
Easy and secure password generator that's completely free and safe to use. Generate strong passwords for every online account with the strong Bitwarden password generator, and get the latest best practices on how to maintain password security and privacy online.

I have a button called BTNPassGenerator, when the user clicks on this ideally the following process needs to be done
> Open website https://bitwarden.com/password-generator (in edge or chrome)
>Type - Passphrase
> Words: 3
> Capitalize - YES
> Include Number - YES
Once this is done then copies the password and Pastes into back into the Access textbox TXTPassword
Thanks in advance!
Stu